Nested Forms in Angular
While I was working with Angular (v19) reactive forms, I came across the need to extract the address into a custom component and reuse it in different forms in order to avoid code duplication. Sounds simple! It is simple indeed, but if you know exactly what you are doing. I've done my research in Google and ended it up on the following credible resource Angular Custom Form Controls: Complete Guide
After reading the article I was about to ditch the idea of a custom component for the Address as the solution wasn't that straightforward, particularly you have to implement ControlValueAccessor and Validator interfaces, add new providers (NG_VALUE_ACCESSOR and NG_VALIDATORS), take care of the Subscriptions to name a few. Fortunately, there is a cleaner way to do it which I described below.
I've created a personal-details component which contains the address component along with first name and last name fields.
As you can see, we are passing the form to an input parameter of the address component. So we have to initialize our form inside OnInit lifecycle hook. Note that we defined address element as a nested form group.
Now let's see what the constituents of the address component are. Nothing interesting in the html - just a form with 3 text fields for city, address and post code.
The interesting part happens behind the scene where we inject ControlContainer in the constructor and later set the addressForm value using it.
In this particular sample you don't need to decorate controlContainer in the constructor with @Optional() and @SkipSelf(), but for more complex scenarios consider doing that.
And finally, to achieve seamless validation of our address component along with other elements from its parent form, we need to call markAllAsTouched() method on the form when the for has an invalid state.
When we click the submit button without populating any data on the form, then all the fields on the form would be displayed in red since we marked all the form elements as required fields.
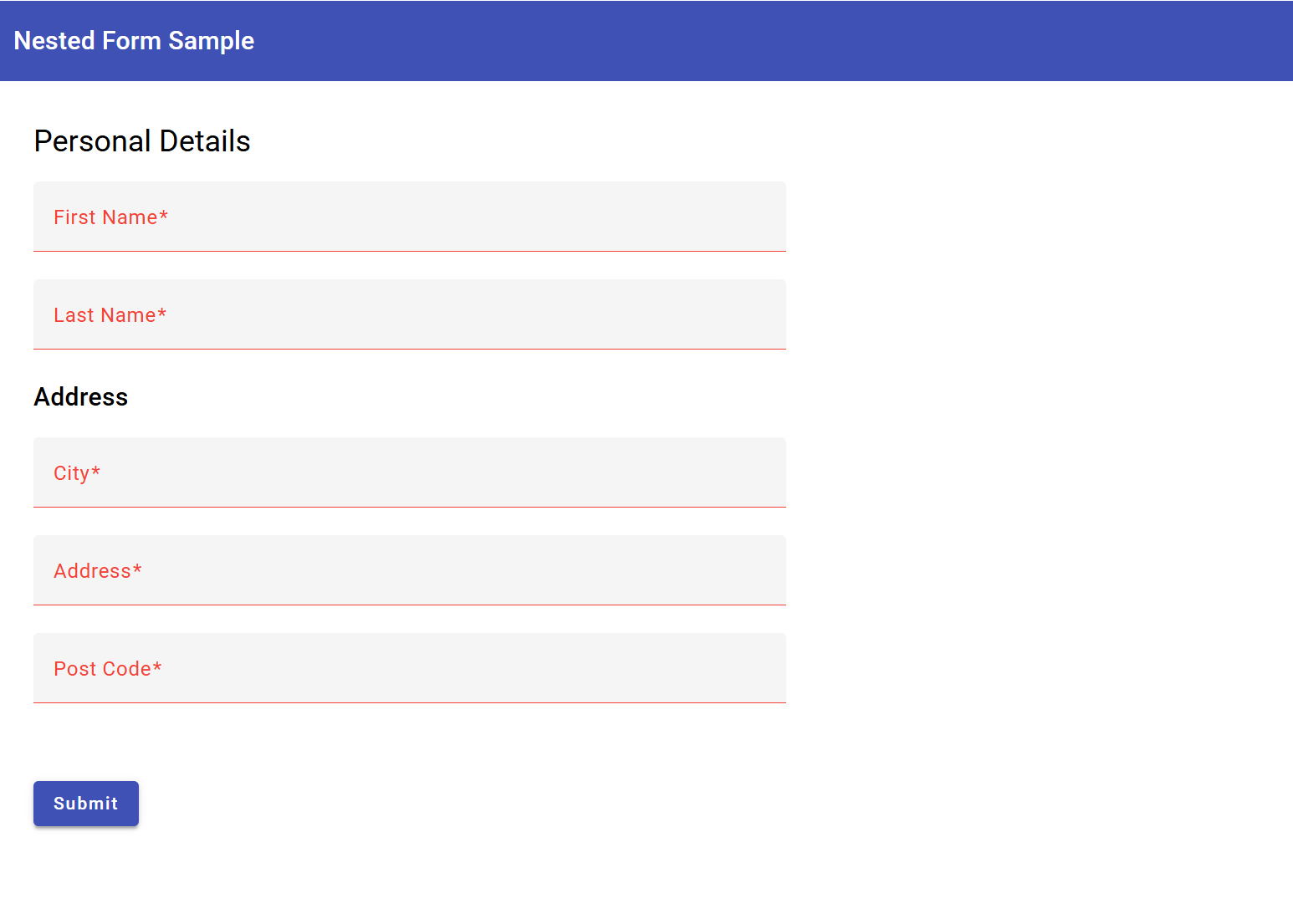
Summary
In this post I illustrated a custom component that could be used in a nested form by leveraging ControlContainer. The source code is available on my Github account.
Happy Coding!