Using GraphQL in .NET ecosystem
GraphQL could be seen as an alternative to REST API. It’s been invented by Facebook in 2012 and now it is adopted by such giants as Twitter and Github. I am not going to discuss the benefits of GraphQL or its difference with REST API. Instead, I will focus on how you can apply GraphQL queries in practice using .NET ecosystem.
This post is split into two parts. In part one we will setup the database, and in second – we will implement GraphQL query.
The natural choice of IDE is Visual Studio. The following technical stack is used in the demo project:
- .NET 5
- Sql Server
- Entity Framework Core
- GraphQL API
- Windows Terminal or other CLI
Also, you will need .NET Core CLI and Entity Framework Core tools to be installed on your machine. Below links would be helpful if you need to install them:
https://docs.microsoft.com/en-us/dotnet/core/sdk
https://docs.microsoft.com/en-us/ef/core/cli/dotnet
For those who like to jump straight to the code – here is the source link:
https://github.com/armache/AM.GraphQL
PART 1 – Database Setup
Let’s start by creating an app using ASP.NET Core Empty template. Run the below command in a CLI:
Add Entity Framework Core dependencies using Nuget or CLI:
Next, we are going to create a simple database using the Code First approach. The database will hold names of the Real Madrid FC players (Los Blancos). Below is the code for PlayerContext.cs
Note: parameterless constructor is needed for the initial migration
Now we are ready to initialize the database, table and 2 sample records. Navigate to your project folder in a CLI and run the following Entity Framework commands:
At this point PlayersDB database should be created in your Sql Server. Switch to Visual Studio and create a IPlayerRepository interface and the class that implements it. The interface would contain a single method – GetPlayers()
Every time when I start the project, I’d like to start with the fresh database. So, I modified the code in Program.cs to delete and recreate the expected database:
PART 2 – GraphQL implementation
It’s time to introduce GraphQL. Add the following dependencies to the project:
Next, we will need to create PlayerType, PlayerQuery, and PlayerSchema classes.
In PlayerType class we do the mapping, so that GraphQL can serialize Player model.
Notice the ListGraphType
Schema describes clients what they can do with an API. In this case they can only query players.
Eventually, we are ready to do the plumbing in Startup.cs
And as a final step, go to the project’s properties, open Debug view, tick “Launch browser”, specify “ui/playground” (without quotes) as the value and save the changes.
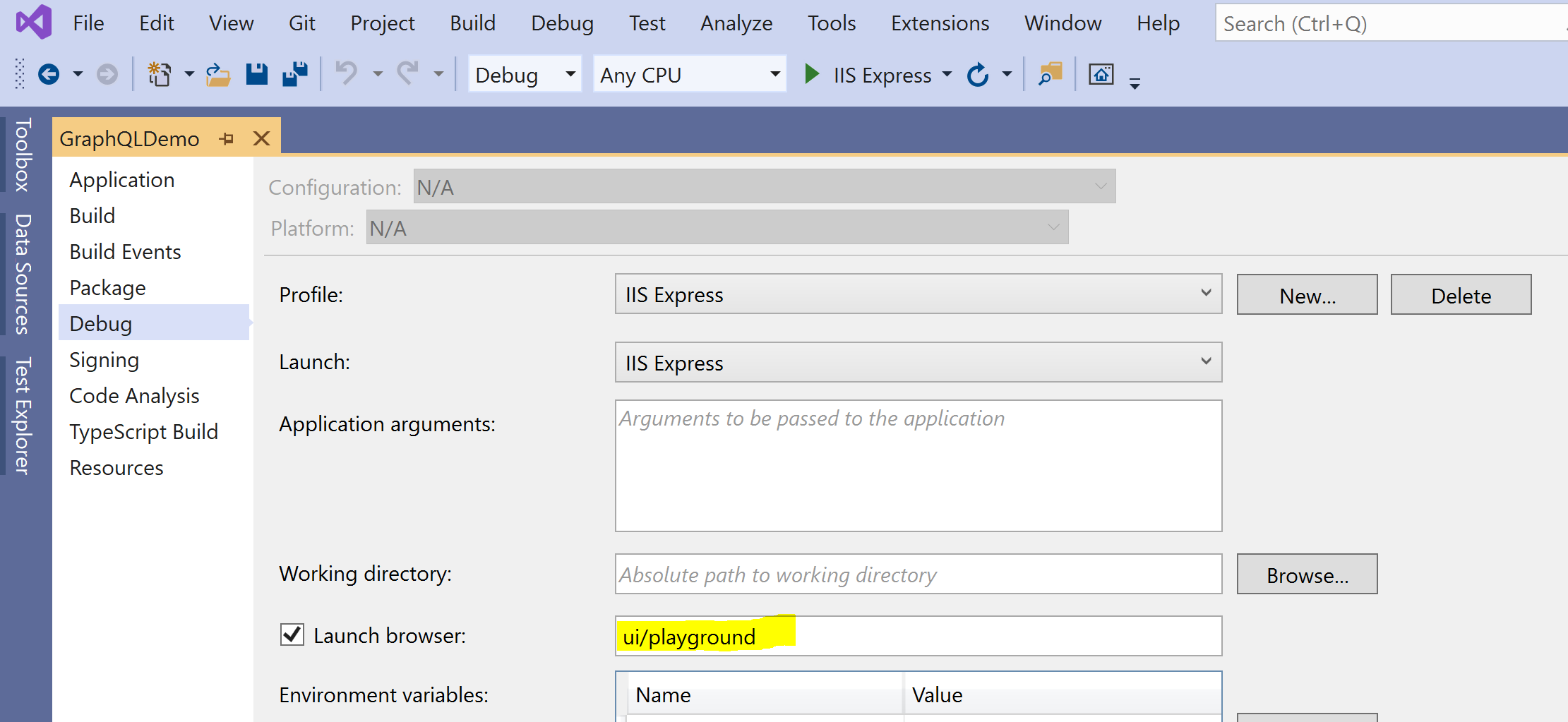
Now start the application Ctrl + F5 and you should see the playground.
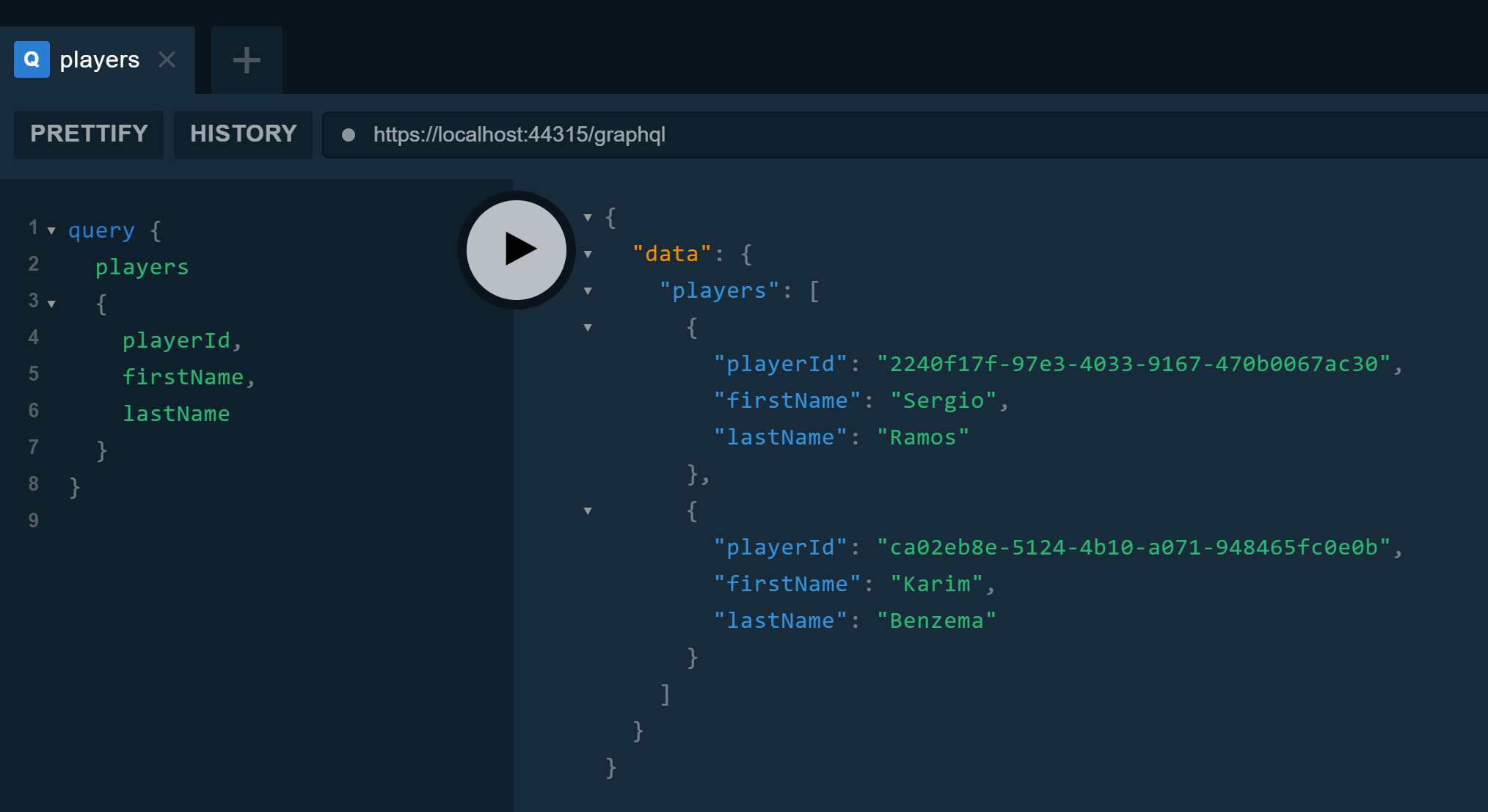
In case you would like to test queries using Postman, Fiddler or any other tool, then you should make a POST request and specify the query in the body of the request in a form of JSON.
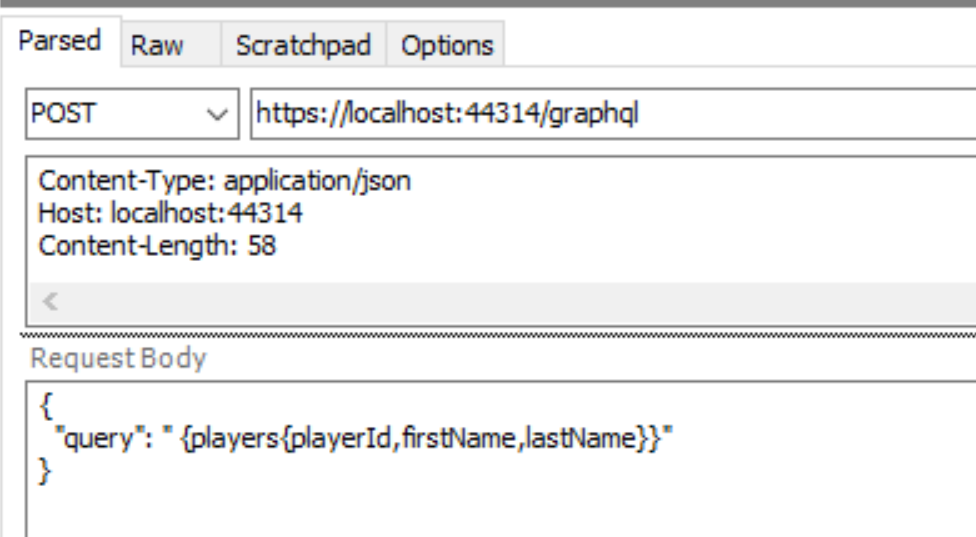
GraphQL always returns 200 OK response. You should check whether the response has errors in the response body. Also, by default response will include “extensions” – which is good for testing, but not the production environment. In our case we switched it off by setting EnableMetrics option to false in ConfigureServices method.
Summary
This post demonstrates both – how to setup SQL Server database using Code First approach and how to apply GraphQL to query the database.
Now you have a choice of technologies for API design, whichever works best would depend on requirements. In order to further demystify GraphQL and get better understanding of its components you can check the links below.
https://graphql.org/learn/https://www.graphql.com/guides/